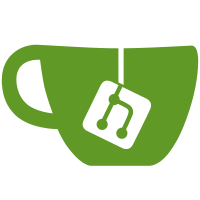
Correctly detect Raspberry Pi 3 on Kernel 4.9
embd

embd is a hardware abstraction layer (HAL) for embedded systems.
It allows you to start your hardware hack on easily available hobby boards (like the Raspberry Pi, BeagleBone Black, C.H.I.P., etc.) by giving you straight-forward access to the board's capabilities as well as a plethora of sensors (like accelerometers, gyroscopes, thermometers, etc.) and controllers (PWM generators, digital-to-analog convertors) for which it includes drivers. If you move to custom designed boards you have to throw away your code: you carry forward the effort where the HAL abstraction of EMBD will save you precious time.
The overall strategy used in embd is to use Linux device drivers to access gpio pins, SPI and I2C buses, as well as interrupts. This makes it easy to port from one platform to another and it enables kernel code to handle the devices as efficiently as possible. What embd then adds is first a Golang library interface on top of the various Linux devices and then another layer of user-level drivers for specific sensors and controllers that are connected to gpio pins or one of the buses.
Development supported and sponsored by SoStronk and ThoughtWorks.
Also, you might be interested in: Why Golang?
Getting Started
Install Go version 1.6 or later to make compiling for ARM easy.
The set up your GOPATH,
and create your first .go file. We'll call it simpleblinker.go
.
package main
import (
"time"
"github.com/kidoman/embd"
_ "github.com/kidoman/embd/host/rpi" // This loads the RPi driver
)
func main() {
for {
embd.LEDToggle("LED0")
time.Sleep(250 * time.Millisecond)
}
}
Then install the EMBD package:
$ go get github.com/kidoman/embd
Build the binary for linux/ARM:
$ export GOOS=linux
$ export GOARCH=arm
$ go build simpleblinker.go
Copy the cross-compiled binary to your RaspberryPi*:
$ scp simpleblinker pi@192.168.2.2:~
Then on the rPi run the program with sudo
*:
$ sudo ./simpleblinker
You will now see the green LED (next to the always on power LED) blink every 1/4 sec.
* Notes
- Assuming your RaspberryPi has an IP address of
192.168.2.2
. Substitute as necessary sudo
(root) permission is required as we are controlling the hardware by writing to special files- This sample program is optimized for brevity and does not clean up after itself. Click here to see the full version
Getting Help
Join the slack channel
Platforms Supported
- RaspberryPi (including A+ and B+)
- RaspberryPi 2
- NextThing C.H.I.P
- BeagleBone Black
The command line tool
go get github.com/kidoman/embd/embd
will install a command line utility embd
which will allow you to quickly get started with prototyping. The binary should be available in your $GOPATH/bin
. However, to be able to run this on a ARM based device, you will need to build it with GOOS=linux
and GOARCH=arm
environment variables set.
For example, if you run embd detect
on a BeagleBone Black:
root@beaglebone:~# embd detect
detected host BeagleBone Black (rev 0)
Run embd
without any arguments to discover the various commands supported by the utility.
How to use the framework
Package embd provides a hardware abstraction layer for doing embedded programming on supported platforms like the Raspberry Pi and BeagleBone Black. Most of the examples below will work without change (i.e. the same binary) on all supported platforms. How cool is that?
Although samples are all present in the samples folder, we will show a few choice examples here.
Use the LED driver to toggle LEDs on the BBB:
import "github.com/kidoman/embd"
import _ "github.com/kidoman/embd/host/all"
...
embd.InitLED()
defer embd.CloseLED()
...
led, err := embd.NewLED(3)
...
led.Toggle()
Even shorter when quickly trying things out:
import "github.com/kidoman/embd"
import _ "github.com/kidoman/embd/host/all"
...
embd.InitLED()
defer embd.CloseLED()
...
embd.ToggleLED(3)
3 is the same as USR3 for all intents and purposes. The driver is smart enough to figure all this out.
BBB + PWM:
import "github.com/kidoman/embd"
import _ "github.com/kidoman/embd/host/all"
...
embd.InitGPIO()
defer embd.CloseGPIO()
...
pwm, _ := embd.NewPWMPin("P9_14")
defer pwm.Close()
...
pwm.SetDuty(1000)
Control GPIO pins on the RaspberryPi / BeagleBone Black:
import "github.com/kidoman/embd"
import _ "github.com/kidoman/embd/host/all"
...
embd.InitGPIO()
defer embd.CloseGPIO()
...
embd.SetDirection(10, embd.Out)
embd.DigitalWrite(10, embd.High)
Could also do:
import "github.com/kidoman/embd"
import _ "github.com/kidoman/embd/host/all"
...
embd.InitGPIO()
defer embd.CloseGPIO()
...
pin, err := embd.NewDigitalPin(10)
...
pin.SetDirection(embd.Out)
pin.Write(embd.High)
Or read data from the Bosch BMP085 barometric sensor:
import "github.com/kidoman/embd"
import "github.com/kidoman/embd/sensor/bmp085"
import _ "github.com/kidoman/embd/host/all"
...
bus := embd.NewI2CBus(1)
...
baro := bmp085.New(bus)
...
temp, err := baro.Temperature()
altitude, err := baro.Altitude()
Even find out the heading from the LSM303 magnetometer:
import "github.com/kidoman/embd"
import "github.com/kidoman/embd/sensor/lsm303"
import _ "github.com/kidoman/embd/host/all"
...
bus := embd.NewI2CBus(1)
...
mag := lsm303.New(bus)
...
heading, err := mag.Heading()
The above two examples depend on I2C and therefore will work without change on almost all platforms.
Protocols Supported
- Digital GPIO Documentation
- Analog GPIO Documentation
- PWM Documentation
- I2C Documentation
- LED Documentation
- SPI Documentation
Sensors Supported
- TMP006 Thermopile sensor Documentation, Datasheet, Userguide
- BMP085 Barometric pressure sensor Documentation, Datasheet
- BMP180 Barometric pressure sensor Documentation, Datasheet
- LSM303 Accelerometer and magnetometer Documentation, Datasheet
- L3GD20 Gyroscope Documentation, Datasheet
- US020 Ultrasonic proximity sensor Documentation, Product Page
- BH1750FVI Luminosity sensor Documentation, Datasheet
Interfaces
- Keypad(4x3) Product Page
Controllers
- PCA9685 16-channel, 12-bit PWM Controller with I2C protocol Documentation, Datasheet, Product Page
- MCP4725 12-bit DAC Documentation, Datasheet, Product Page
- ServoBlaster RPi PWM/PCM based PWM controller Documentation, Product Page
Convertors
- MCP3008 8-channel, 10-bit ADC with SPI protocol, Datasheet
Contributing
Pull requests that follow the guidelines are very appreciated. If you find a problem but are not up to coding a fix please file an issue. Thank you!
About
EMBD is affectionately designed/developed by Karan Misra (kidoman), Kunal Powar (kunalpowar) and FRIENDS. We also have a list of CONTRIBUTORS.