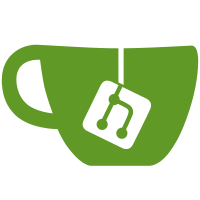
the us020 driver is the first consumer of this functionality
embd

A superheroic hardware abstraction layer for doing embedded programming on supported platforms like the Raspberry Pi and BeagleBone Black.
Development sponsored by ThoughtWorks
Platforms supported
- RaspberryPi
- BeagleBone Black
- Intel Galileo coming soon
- Radxa coming soon
- Cubietruck coming soon
- Bring Your Own coming soon
How to use
Package embd provides a superheroic hardware abstraction layer for doing embedded programming on supported platforms like the Raspberry Pi and BeagleBone Black. Most of the examples below will work without change (i.e. the same binary) on all supported platforms. How cool is that?
Although samples are all present in the samples folder, we will show a few choice examples here.
Use the LED driver to toggle LEDs on the BBB:
import "github.com/kidoman/embd"
...
embd.InitLED()
defer embd.CloseLED()
...
led, err := embd.NewLED("USR3")
...
led.Toggle()
Even shorter while prototyping:
import "github.com/kidoman/embd"
...
embd.InitLED()
defer embd.CloseLED()
...
embd.ToggleLED(3)
NB: 3 == USR3 for all intents and purposes. The driver is smart enough to figure all this out.
BBB + PWM:
import "github.com/kidoman/embd"
...
embd.InitGPIO()
defer embd.CloseGPIO()
...
pwm, _ := embd.NewPWMPin("P9_14")
defer pwm.Close()
...
pwm.SetDuty(1000)
Control GPIO pins on the RaspberryPi / BeagleBone Black:
import "github.com/kidoman/embd"
...
embd.InitGPIO()
defer embd.CloseGPIO()
...
embd.SetDirection(10, embd.Out)
embd.DigitalWrite(10, embd.High)
Could also do:
import "github.com/kidoman/embd"
...
embd.InitGPIO()
defer embd.CloseGPIO()
...
pin, err := embd.NewDigitalPin(10)
...
pin.SetDirection(embd.Out)
pin.Write(embd.High)
Or read data from the Bosch BMP085 barometric sensor:
import "github.com/kidoman/embd"
import "github.com/kidoman/embd/sensor/bmp085"
...
bus := embd.NewI2CBus(1)
...
baro := bmp085.New(bus)
...
temp, err := baro.Temperature()
altitude, err := baro.Altitude()
Even find out the heading from the LSM303 magnetometer:
import "github.com/kidoman/embd"
import "github.com/kidoman/embd/sensor/lsm303"
...
bus := embd.NewI2CBus(1)
...
mag := lsm303.New(bus)
...
heading, err := mag.Heading()
The above two examples depend on I2C and therefore will work without change on almost all platforms.
Protocols supported
- Digital GPIO Documentation
- Analog GPIO Documentation
- PWM Documentation
- I2C Documentation
- LED Documentation
Sensors supported
-
TMP006 Thermopile sensor Documentation, Datasheet, Userguide
-
BMP085 Barometric pressure sensor Documentation, Datasheet
-
BMP180 Barometric pressure sensor Documentation, Datasheet
-
LSM303 Accelerometer and magnetometer Documentation, Datasheet
-
L3GD20 Gyroscope Documentation, Datasheet
-
US020 Ultrasonic proximity sensor Documentation, Product Page
-
BH1750FVI Luminosity sensor Documentation, Datasheet
Interfaces
- Keypad(4x3) Product Page
Controllers
-
PCA9685 16-channel, 12-bit PWM Controller with I2C protocol Documentation, Datasheet, Product Page
-
MCP4725 12-bit DAC Documentation, Datasheet, Product Page
-
ServoBlaster RPi PWM/PCM based PWM controller Documentation, Product Page