mirror of
https://github.com/meilisearch/MeiliSearch
synced 2024-11-05 12:38:55 +01:00
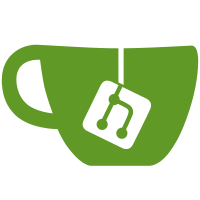
- `check_tag`: Report file name correctly. Use named variables. - Introduce `read_version` helper function. Simplify the implementation. - Show meaningful error message if `GITHUB_REF` is not set or its format is incorrect.
42 lines
955 B
Bash
42 lines
955 B
Bash
#!/usr/bin/env bash
|
|
set -eu -o pipefail
|
|
|
|
check_tag() {
|
|
local expected=$1
|
|
local actual=$2
|
|
local filename=$3
|
|
|
|
if [[ $actual != $expected ]]; then
|
|
echo >&2 "Error: the current tag does not match the version in $filename: found $actual, expected $expected"
|
|
return 1
|
|
fi
|
|
}
|
|
|
|
read_version() {
|
|
grep '^version = ' | cut -d \" -f 2
|
|
}
|
|
|
|
if [[ -z "${GITHUB_REF:-}" ]]; then
|
|
echo >&2 "Error: GITHUB_REF is not set"
|
|
exit 1
|
|
fi
|
|
|
|
if [[ ! "$GITHUB_REF" =~ ^refs/tags/v[0-9]+\.[0-9]+\.[0-9]+(-[a-z0-9]+)?$ ]]; then
|
|
echo >&2 "Error: GITHUB_REF is not a valid tag: $GITHUB_REF"
|
|
exit 1
|
|
fi
|
|
|
|
current_tag=${GITHUB_REF#refs/tags/v}
|
|
ret=0
|
|
|
|
toml_tag="$(cat Cargo.toml | read_version)"
|
|
check_tag "$current_tag" "$toml_tag" Cargo.toml || ret=1
|
|
|
|
lock_tag=$(grep -A 1 '^name = "meilisearch-auth"' Cargo.lock | read_version)
|
|
check_tag "$current_tag" "$lock_tag" Cargo.lock || ret=1
|
|
|
|
if (( ret == 0 )); then
|
|
echo 'OK'
|
|
fi
|
|
exit $ret
|