mirror of
https://github.com/meilisearch/MeiliSearch
synced 2025-07-04 20:37:15 +02:00
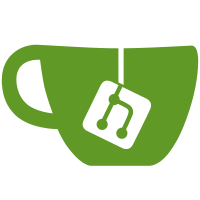
I have an issue where "speakers" is split into "speaker" and "s", when I compute the distances for the Typo criterion, it takes "s" into account and put a distance of zero in the bucket 0 (the "speakers" bucket), therefore it reports any document matching "s" without typos as best results. I need to make sure to ignore "s" when its associated part "speaker" doesn't even exist in the document and is not in the place it should be ("speaker" followed by "s"). This is hard to think that it will had much computation time to the Typo criterion like in the previous algorithm where I computed the real query/words indexes based and removed the invalid ones before sending the documents to the bucket sort.
53 lines
1.6 KiB
Rust
53 lines
1.6 KiB
Rust
use levenshtein_automata::{LevenshteinAutomatonBuilder as LevBuilder, DFA};
|
|
use once_cell::sync::OnceCell;
|
|
|
|
static LEVDIST0: OnceCell<LevBuilder> = OnceCell::new();
|
|
static LEVDIST1: OnceCell<LevBuilder> = OnceCell::new();
|
|
static LEVDIST2: OnceCell<LevBuilder> = OnceCell::new();
|
|
|
|
#[derive(Copy, Clone)]
|
|
enum PrefixSetting {
|
|
Prefix,
|
|
NoPrefix,
|
|
}
|
|
|
|
fn build_dfa_with_setting(query: &str, setting: PrefixSetting) -> DFA {
|
|
use PrefixSetting::{NoPrefix, Prefix};
|
|
|
|
match query.len() {
|
|
0..=4 => {
|
|
let builder = LEVDIST0.get_or_init(|| LevBuilder::new(0, true));
|
|
match setting {
|
|
Prefix => builder.build_prefix_dfa(query),
|
|
NoPrefix => builder.build_dfa(query),
|
|
}
|
|
}
|
|
5..=8 => {
|
|
let builder = LEVDIST1.get_or_init(|| LevBuilder::new(1, true));
|
|
match setting {
|
|
Prefix => builder.build_prefix_dfa(query),
|
|
NoPrefix => builder.build_dfa(query),
|
|
}
|
|
}
|
|
_ => {
|
|
let builder = LEVDIST2.get_or_init(|| LevBuilder::new(2, true));
|
|
match setting {
|
|
Prefix => builder.build_prefix_dfa(query),
|
|
NoPrefix => builder.build_dfa(query),
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
pub fn build_prefix_dfa(query: &str) -> DFA {
|
|
build_dfa_with_setting(query, PrefixSetting::Prefix)
|
|
}
|
|
|
|
pub fn build_dfa(query: &str) -> DFA {
|
|
build_dfa_with_setting(query, PrefixSetting::NoPrefix)
|
|
}
|
|
|
|
pub fn build_exact_dfa(query: &str) -> DFA {
|
|
let builder = LEVDIST0.get_or_init(|| LevBuilder::new(0, true));
|
|
builder.build_dfa(query)
|
|
}
|