mirror of
git://git.gnupg.org/gnupg.git
synced 2024-05-29 21:58:04 +02:00
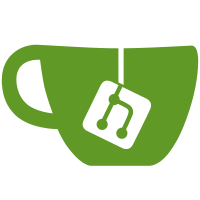
* kbx/keybox-util.c (keybox_set_malloc_hooks): Remove. (_keybox_malloc, _keybox_calloc, keybox_realloc) (_keybox_free): Remove. (keybox_file_rename): Remove. Was not used. * sm/gpgsm.c (main): Remove call to keybox_set_malloc_hooks. * kbx/kbxutil.c (main): Ditto. * kbx/keybox-defs.h: Remove all separate includes. Include util.h. remove convenience macros. * common/logging.h (return_if_fail): New. Originally from keybox-defs.h but now using log_debug. (return_null_if_fail): Ditto. (return_val_if_fail): Ditto. (never_reached): Ditto. -- Originally the KBX code was written to allow standalone use. However this required lot of ugliness like separate memory allocators and such. It also precludes the use of some standard functions from common due to their use of the common gnupg malloc functions. Dropping all that makes things easier. Minor disadvantages: the kbx call done for gpg will now use gcry malloc fucntions and not the standard malloc functions. This might be a bit slower but removing them even fixes a possible bug in keybox_tmp_names which is used in gpg and uses gpg's xfree which is actually gcry_free. Signed-off-by: Werner Koch <wk@gnupg.org>
103 lines
3.4 KiB
C
103 lines
3.4 KiB
C
/* keybox-util.c - Utility functions for Keybox
|
|
* Copyright (C) 2001 Free Software Foundation, Inc.
|
|
*
|
|
* This file is part of GnuPG.
|
|
*
|
|
* GnuPG is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* GnuPG is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <https://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <config.h>
|
|
#include <stdlib.h>
|
|
#include <stdio.h>
|
|
#include <string.h>
|
|
#ifdef HAVE_DOSISH_SYSTEM
|
|
# define WIN32_LEAN_AND_MEAN /* We only need the OS core stuff. */
|
|
# include <windows.h>
|
|
#endif
|
|
|
|
#include "keybox-defs.h"
|
|
|
|
|
|
/* Store the two malloced temporary file names used for keybox updates
|
|
of file FILENAME at R_BAKNAME and R_TMPNAME. On error an error
|
|
code is returned and NULL stored at R_BAKNAME and R_TMPNAME. If
|
|
FOR_KEYRING is true the returned names match those used by GnuPG's
|
|
keyring code. */
|
|
gpg_error_t
|
|
keybox_tmp_names (const char *filename, int for_keyring,
|
|
char **r_bakname, char **r_tmpname)
|
|
{
|
|
gpg_error_t err;
|
|
char *bak_name, *tmp_name;
|
|
|
|
*r_bakname = NULL;
|
|
*r_tmpname = NULL;
|
|
|
|
# ifdef USE_ONLY_8DOT3
|
|
/* Here is another Windoze bug?:
|
|
* you can't rename("pubring.kbx.tmp", "pubring.kbx");
|
|
* but rename("pubring.kbx.tmp", "pubring.aaa");
|
|
* works. So we replace ".kbx" by ".kb_" or ".k__". Note that we
|
|
* can't use ".bak" and ".tmp", because these suffixes are used by
|
|
* gpg's keyrings and would lead to a sharing violation or data
|
|
* corruption. If the name does not end in ".kbx" we assume working
|
|
* on a modern file system and append the suffix. */
|
|
{
|
|
const char *ext = for_keyring? EXTSEP_S GPGEXT_GPG : EXTSEP_S "kbx";
|
|
const char *b_ext = for_keyring? EXTSEP_S "bak" : EXTSEP_S "kb_";
|
|
const char *t_ext = for_keyring? EXTSEP_S "tmp" : EXTSEP_S "k__";
|
|
int repl;
|
|
|
|
if (strlen (ext) != 4 || strlen (b_ext) != 4)
|
|
BUG ();
|
|
repl = (strlen (filename) > 4
|
|
&& !strcmp (filename + strlen (filename) - 4, ext));
|
|
bak_name = xtrymalloc (strlen (filename) + (repl?0:4) + 1);
|
|
if (!bak_name)
|
|
return gpg_error_from_syserror ();
|
|
strcpy (bak_name, filename);
|
|
strcpy (bak_name + strlen (filename) - (repl?4:0), b_ext);
|
|
|
|
tmp_name = xtrymalloc (strlen (filename) + (repl?0:4) + 1);
|
|
if (!tmp_name)
|
|
{
|
|
err = gpg_error_from_syserror ();
|
|
xfree (bak_name);
|
|
return err;
|
|
}
|
|
strcpy (tmp_name, filename);
|
|
strcpy (tmp_name + strlen (filename) - (repl?4:0), t_ext);
|
|
}
|
|
# else /* Posix file names */
|
|
(void)for_keyring;
|
|
bak_name = xtrymalloc (strlen (filename) + 2);
|
|
if (!bak_name)
|
|
return gpg_error_from_syserror ();
|
|
strcpy (stpcpy (bak_name, filename), "~");
|
|
|
|
tmp_name = xtrymalloc (strlen (filename) + 5);
|
|
if (!tmp_name)
|
|
{
|
|
err = gpg_error_from_syserror ();
|
|
xfree (bak_name);
|
|
return err;
|
|
}
|
|
strcpy (stpcpy (tmp_name,filename), EXTSEP_S "tmp");
|
|
# endif /* Posix filename */
|
|
|
|
*r_bakname = bak_name;
|
|
*r_tmpname = tmp_name;
|
|
return 0;
|
|
}
|