mirror of
git://git.gnupg.org/gnupg.git
synced 2024-10-31 20:08:43 +01:00
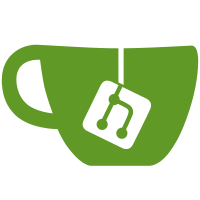
* common/dns-cert.c: Remove iobuf.h. (get_dns_cert): Rename to _get_dns_cert. Remove MAX_SIZE arg. Change iobuf arg to a estream-t. Rewrite function to make use of estream instead of iobuf. Require all parameters. Return an gpg_error_t error instead of the type. Add arg ERRSOURCE. * common/dns-cert.h (get_dns_cert): New macro to pass the error source to _gpg_dns_cert. * common/t-dns-cert.c (main): Adjust for changes in get_dns_cert. * g10/keyserver.c (keyserver_import_cert): Ditto. * doc/gpg.texi (GPG Configuration Options): Remove max-cert-size.
96 lines
2.0 KiB
C
96 lines
2.0 KiB
C
/* t-dns-cert.c - Module test for dns-cert.c
|
|
* Copyright (C) 2011 Free Software Foundation, Inc.
|
|
*
|
|
* This file is part of GnuPG.
|
|
*
|
|
* GnuPG is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* GnuPG is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <config.h>
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <assert.h>
|
|
|
|
#include "util.h"
|
|
#include "dns-cert.h"
|
|
|
|
|
|
int
|
|
main (int argc, char **argv)
|
|
{
|
|
gpg_error_t err;
|
|
unsigned char *fpr;
|
|
size_t fpr_len;
|
|
char *url;
|
|
estream_t key;
|
|
char const *name;
|
|
|
|
if (argc)
|
|
{
|
|
argc--;
|
|
argv++;
|
|
}
|
|
|
|
if (!argc)
|
|
name = "simon.josefsson.org";
|
|
else if (argc == 1)
|
|
name = *argv;
|
|
else
|
|
{
|
|
fputs ("usage: t-dns-cert [name]\n", stderr);
|
|
return 1;
|
|
}
|
|
|
|
printf ("CERT lookup on `%s'\n", name);
|
|
|
|
err = get_dns_cert (name, &key, &fpr, &fpr_len, &url);
|
|
if (err)
|
|
printf ("get_dns_cert failed: %s <%s>\n",
|
|
gpg_strerror (err), gpg_strsource (err));
|
|
else if (key)
|
|
{
|
|
int count = 0;
|
|
|
|
while (es_getc (key) != EOF)
|
|
count++;
|
|
printf ("Key found (%d bytes)\n", count);
|
|
}
|
|
else
|
|
{
|
|
if (fpr)
|
|
{
|
|
int i;
|
|
|
|
printf ("Fingerprint found (%d bytes): ", (int)fpr_len);
|
|
for (i = 0; i < fpr_len; i++)
|
|
printf ("%02X", fpr[i]);
|
|
putchar ('\n');
|
|
}
|
|
else
|
|
printf ("No fingerprint found\n");
|
|
|
|
if (url)
|
|
printf ("URL found: %s\n", url);
|
|
else
|
|
printf ("No URL found\n");
|
|
|
|
}
|
|
|
|
es_fclose (key);
|
|
xfree (fpr);
|
|
xfree (url);
|
|
|
|
return 0;
|
|
}
|