mirror of
git://git.gnupg.org/gnupg.git
synced 2024-06-02 22:38:02 +02:00
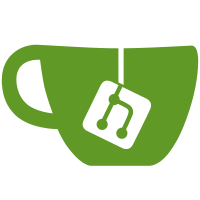
* common/estream-printf.c (_ESTREAM_PRINTF_MALLOC): Remove. (_ESTREAM_PRINTF_FREE): Remove. (_ESTREAM_PRINTF_REALLOC): New. (fixed_realloc) [!_ESTREAM_PRINTF_REALLOC]): New. (estream_vasprintf): Use my_printf_realloc instead of my_printf_malloc and my_printf_free. (dynamic_buffer_out): Use my_printf_realloc instead of realloc. -- This bug will never happen in current GnuPG/Libgcrypt because we use the standard memory allocation functions via Libgcrypt. However, when used in other environments it would mess up the heap for an asprintf with an output length larger than ~512 bytes.
62 lines
1.6 KiB
C
62 lines
1.6 KiB
C
/* xasprintf.c
|
|
* Copyright (C) 2003, 2005 Free Software Foundation, Inc.
|
|
*
|
|
* This file is part of GnuPG.
|
|
*
|
|
* GnuPG is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* GnuPG is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <config.h>
|
|
#include <stdlib.h>
|
|
#include <errno.h>
|
|
|
|
#include "util.h"
|
|
#include "iobuf.h"
|
|
|
|
#if !defined(_ESTREAM_PRINTF_REALLOC)
|
|
#error Need to define _ESTREAM_PRINTF_REALLOC
|
|
#endif
|
|
|
|
/* Same as asprintf but return an allocated buffer suitable to be
|
|
freed using xfree. This function simply dies on memory failure,
|
|
thus no extra check is required. */
|
|
char *
|
|
xasprintf (const char *fmt, ...)
|
|
{
|
|
va_list ap;
|
|
char *buf;
|
|
|
|
va_start (ap, fmt);
|
|
if (estream_vasprintf (&buf, fmt, ap) < 0)
|
|
log_fatal ("estream_asprintf failed: %s\n", strerror (errno));
|
|
va_end (ap);
|
|
return buf;
|
|
}
|
|
|
|
/* Same as above but return NULL on memory failure. */
|
|
char *
|
|
xtryasprintf (const char *fmt, ...)
|
|
{
|
|
int rc;
|
|
va_list ap;
|
|
char *buf;
|
|
|
|
va_start (ap, fmt);
|
|
rc = estream_vasprintf (&buf, fmt, ap);
|
|
va_end (ap);
|
|
if (rc < 0)
|
|
return NULL;
|
|
return buf;
|
|
}
|